Everything You Should Know About Promises in JavaScript
Everything You Should Know About Promises in JavaScript
Promises are a programming construct in JavaScript that represent the eventual completion or failure of an asynchronous operation and its resulting value. They are a part of the ECMAScript 6 (ES6) specification and have become a fundamental tool for handling asynchronous code in a more organized and readable manner.
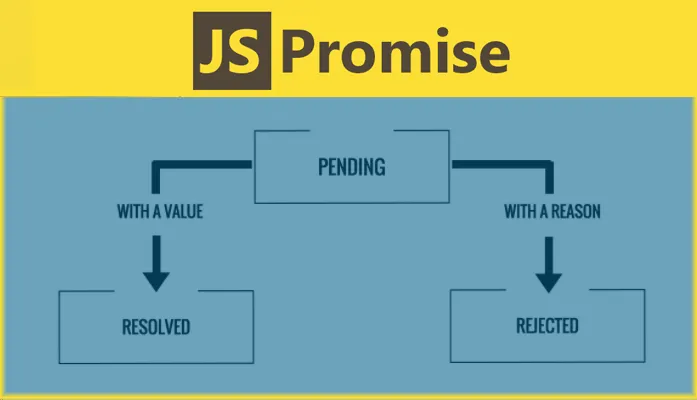
Here are some key points to understand about promises:
Asynchronous Operations:
- Promises are particularly useful for dealing with asynchronous operations, such as fetching data from a server, reading a file, or handling user input.
States:
- A promise can be in one of three states: pending, fulfilled, or rejected.
- When a promise is created, it starts in the pending state.
- If the asynchronous operation is successful, the promise transitions to the fulfilled state with a result value.
- If there is an error during the operation, the promise transitions to the rejected state with a reason for the failure.
Creation:
- Promises are created using the
Promise
constructor. The constructor takes a single argument, a function (executor), which is called immediately and should contain the asynchronous operation.
const myPromise = new Promise((resolve, reject) => {
// Asynchronous operation
// If successful, call resolve(result)
// If there is an error, call reject(error)
});
Consuming Promises:
- Promises are consumed using the
then
andcatch
methods. - The
then
method is used to handle the fulfillment of the promise. - The
catch
method is used to handle any errors that occur during the promise execution.
myPromise
.then(result => {
// Handle successful fulfillment
})
.catch(error => {
// Handle error
});
Chaining:
- Promises can be chained using multiple
then
calls, making it easy to sequence asynchronous operations.
myPromise
.then(result => {
// Do something with the result
return anotherPromise;
})
.then(anotherResult => {
// Handle the result of the second promise
})
.catch(error => {
// Handle errors from any part of the chain
});
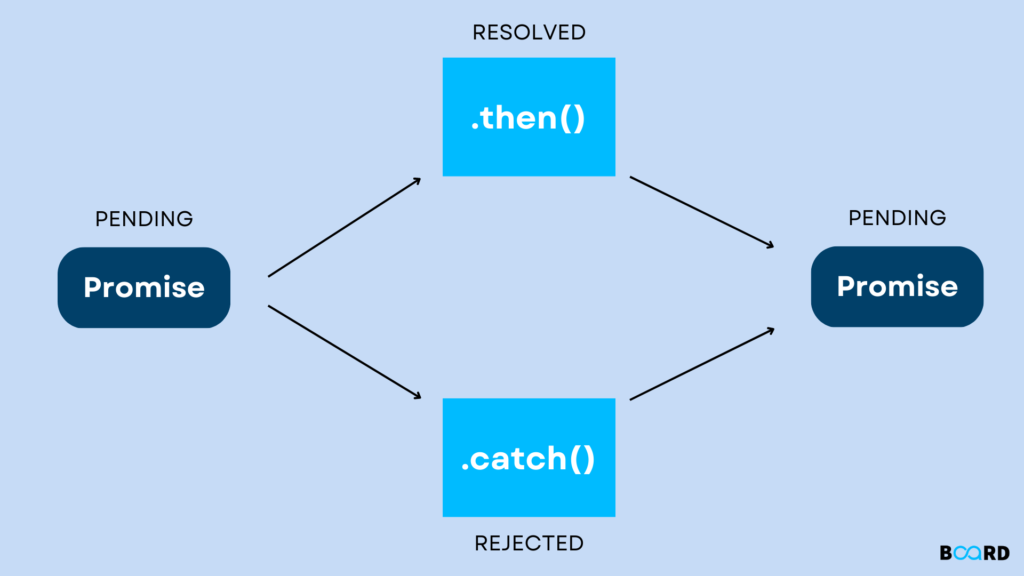
Promise.all and Promise.race:
Promise.all
is used to wait for all promises in an array to fulfill. It returns a promise that fulfills with an array of results.Promise.race
is used to wait for the first promise in an array to fulfill or reject. It returns a promise with the result or reason of the first resolved promise.
const promise1 = fetchData1();
const promise2 = fetchData2();
Promise.all([promise1, promise2])
.then(results => {
// Handle results array
})
.catch(error => {
// Handle errors
});
Async/Await:
- ES6 introduced the
async
andawait
keywords, providing a more concise and synchronous-like way to work with promises.
async function myAsyncFunction() {
try {
const result = await myPromise;
// Do something with the result
} catch (error) {
// Handle errors
}
}
Error Handling:
- Always handle errors in the
catch
block to prevent unhandled promise rejections.
Promises vs Callbacks:
- Promises provide a more structured and readable way to handle asynchronous code compared to traditional callback-based approaches, reducing callback hell (also known as the “pyramid of doom”).
Browser Support:
- Promises are supported in most modern browsers. However, if you need to support older browsers, consider using a polyfill or transpiling your code.
Understanding promises is crucial for writing efficient and maintainable asynchronous code in JavaScript. They play a central role in modern web development, especially when dealing with APIs, network requests, and other asynchronous tasks.
More Examples
// Simulating an asynchronous function to fetch user data
function fetchUsers() {
return new Promise((resolve, reject) => {
// Simulating a delay for asynchronous operation
setTimeout(() => {
const users = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' },
{ id: 3, name: 'Bob Johnson' },
];
// Simulating success
resolve(users);
// Simulating an error (uncomment the line below to test the error case)
// reject(new Error('Failed to fetch users'));
}, 1000); // Simulating a 1-second delay
});
}
// Example of consuming the promise using the then and catch methods
fetchUsers()
.then(users => {
console.log('Users successfully fetched:', users);
// You can perform further operations with the users here
// For example, update the UI, make additional requests, etc.
})
.catch(error => {
console.error('Error fetching users:', error.message);
// Handle the error, such as displaying an error message to the user
});
In this example:
- The
fetchUsers
function returns a promise, simulating an asynchronous operation to fetch user data. - The
then
method is used to handle the successful fulfillment of the promise, where you can work with the fetched users. - The
catch
method is used to handle errors that may occur during the promise execution.
Promise.all Example:
// Simulating an asynchronous function to fetch user data by ID
function fetchUserById(id) {
return new Promise((resolve, reject) => {
// Simulating a delay for asynchronous operation
setTimeout(() => {
const user = users.find(u => u.id === id);
if (user) {
resolve(user);
} else {
reject(new Error(`User with ID ${id} not found`));
}
}, 1000); // Simulating a 1-second delay
});
}
// Simulating an array of user IDs to fetch concurrently
const userIds = [1, 2, 3];
// Using Promise.all to fetch user data for multiple users concurrently
Promise.all(userIds.map(fetchUserById))
.then(usersArray => {
console.log('Users successfully fetched using Promise.all:', usersArray);
})
.catch(error => {
console.error('Error fetching users using Promise.all:', error.message);
});
Promise.race Example:
// Simulating an asynchronous function to fetch user data with a delay
function fetchUserWithDelay(user, delay) {
return new Promise(resolve => {
setTimeout(() => {
resolve(user);
}, delay);
});
}
// Using Promise.race to fetch the user who responds first
const user1Promise = fetchUserWithDelay({ id: 1, name: 'John Doe' }, 2000); // 2 seconds delay
const user2Promise = fetchUserWithDelay({ id: 2, name: 'Jane Smith' }, 1000); // 1 second delay
Promise.race([user1Promise, user2Promise])
.then(winner => {
console.log('The first user to respond:', winner);
})
.catch(error => {
console.error('Error in Promise.race example:', error.message);
});
Async/Await Example:
// Async function using await to fetch users
async function fetchUsersAsync() {
try {
const users = await fetchUsers();
console.log('Users successfully fetched using async/await:', users);
// You can perform further operations with the users here
// For example, update the UI, make additional requests, etc.
} catch (error) {
console.error('Error fetching users using async/await:', error.message);
// Handle the error, such as displaying an error message to the user
}
}
// Call the async function
fetchUsersAsync();
“JavaScript Promises tutorial for beginners”
“Understanding Promises in JavaScript step by step”
“Easy guide to JavaScript Promises”
“Best practices for using Promises in JavaScript”
“Promises vs Callbacks comparison in JavaScript”
“Handling asynchronous operations with Promises in JS”
“JavaScript Promise resolution and rejection explained”
“Common mistakes with Promises in JavaScript”
“Promises explained in plain language”
“JavaScript Promises cheat sheet for developers”
“Asynchronous programming with Promises in JS”
“JavaScript Promises examples and exercises”
“Promises demystified: A beginner’s guide in JavaScript”
“JavaScript Promises for improved code readability”
“How to avoid callback hell using Promises in JS”